See sb3-ai-sample for a sample project based on this post.
Pre-requisites
OpenAI API Key
- Create an OpenAI Account if you don't already have one.
- Navigate to the API Keys page and create a new API key.
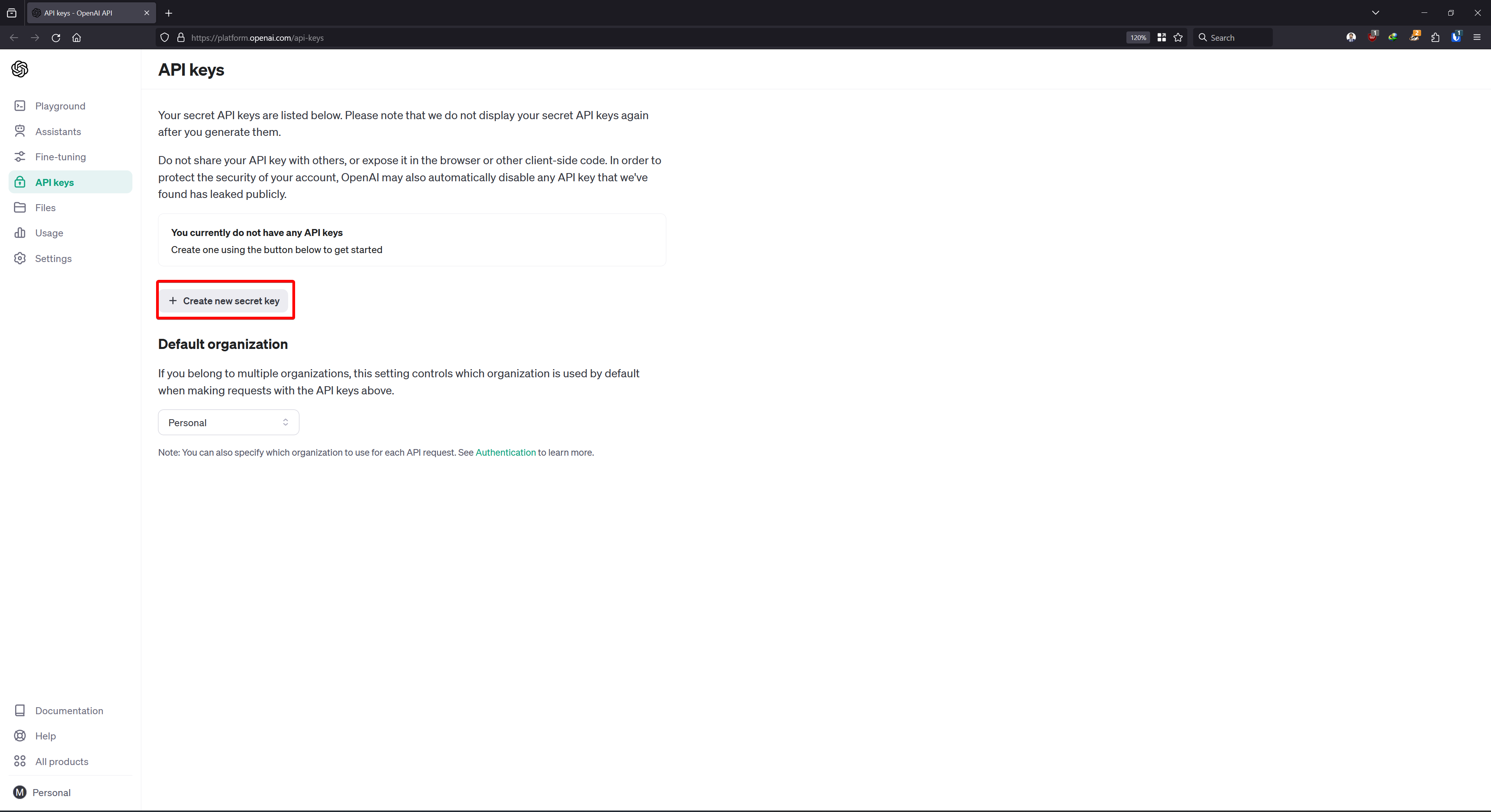
- Save this key for later as it'll be used to set the
SPRING_AI_OPENAI_API_KEY
environment variable.
Note: You will be eligible for some free credits ($5) if you created a new account. However, those credits are only valid for 3 months. Consequently, you'll need to purchase some credits if you want to use the API for longer. Review the Pricing - OpenAI and Rate Limits - OpenAI for more information.
Initialize a new Spring AI project
Use the spring-boot cli to create a new spring-ai project:
#!/usr/bin/env bash
spring boot new ai \
--name . \
--group-id "com.muneebkhawaja.ai" \
--artifact-id "sample" \
--version "0.0.1-SNAPSHOT"
Alternatively, if using PowerShell:
#!/usr/bin/env pwsh
spring.exe boot new ai `
--name . `
--group-id "com.muneebkhawaja.ai" `
--artifact-id "sample" `
--version "0.0.1-SNAPSHOT"
Note: Update the --name <name-of-project>
option if you want to create a new directory for the project.
Your project should have a similar structure to the following:
├── .gitignore
├── README.md
├── mvnw
├── mvnw.cmd
├── pom.xml
└── src
└── main
└── java
└── com
└── muneebkhawaja
└── ai
└── sample
├── Application.java
└── simple
├── Completion.java
└── SimpleAiController.java
Updating Dependencies
At the time of writing, the following dependency is added to the pom.xml
when creating the project via the spring cli i.e.
<dependency>
<groupId>org.springframework.experimental.ai</groupId>
<artifactId>spring-ai-openai-spring-boot-starter</artifactId>
<version>0.2.0-SNAPSHOT</version>
</dependency>
According to the spring-ai project README.md on GitHub, the project's group ids are transitioning from org.springframework.experimental.ai
to org.springframework.ai
. As such, review the Spring AI Documentation for the latest version and update the spring-ai-openai-spring-boot-starter
dependency in the pom.xml
accordingly i.e.
<dependency>
<groupId>org.springframework.ai</groupId>
<artifactId>spring-ai-openai-spring-boot-starter</artifactId>
<version>0.8.0-SNAPSHOT</version>
</dependency>
Running the application
#!/usr/bin/env bash
export SPRING_AI_OPENAI_API_KEY=<INSERT KEY HERE>
./mvnw spring-boot:run
Alternatively, if using PowerShell:
#!/usr/bin/env pwsh
set-content env:SPRING_AI_OPENAI_API_KEY "<INSERT KEY HERE>"
.\mvnw.cmd spring-boot:run
The application should be accessible at http://localhost:8080
Trying out endpoints
The quick start project comes with some default endpoints that you can try out i.e.
#!/usr/bin/env bash
curl --get --data-urlencode 'message=Tell me a joke about a cow.' http://localhost:8080/ai/simple
Alternatively, if using PowerShell:
#!/usr/bin/env pwsh
Invoke-WebRequest -Method Get -Uri http://localhost:8080/ai/simple -Body @{ message = "Tell me a joke about a cow." }
A sample response is given below:
Why did the cow go to space?
Because it wanted to see the mooooon!
Notes about Model Usage
The default implementation of AiClient in spring-ai-openai-spring-boot-starter
i.e. OpenAiClient
uses the gpt-3.5-turbo
model by default.
References
- Pricing - OpenAI, openai.com/pricing
- Rate Limits - OpenAI API, platform.openai.com/docs/guides/rate-limits/usage-tiers
- Spring AI Reference, docs.spring.io/spring-ai/reference
- Spring-CLI, github.com/spring-projects/spring-cli/releases
- Spring-Projects/Spring-AI: An Application Framework for AI Engineering, github.com/spring-projects/spring-ai
- Temurin JDK-17 Releases, adoptium.net/temurin/releases/?package=jdk&version=17
- "What happens after I use my free tokens or the 3 months is up in the free trial", OpenAI
Help, help.openai.com/en/articles/4936830-what-happens-after-i-use-my-free-tokens-or-the-3-months-is-up-in-the-free-trial
Spring AI with OpenAI Models
Dive straight into using Spring AI with Open AI models via the quick start project and explore the integration.